The Arduino board can transmit and receive data to a personal computer over USB cable, this comes handy when you want to send the sensor data from microcontroller to a personal computer.
The Arduino IDE has built-in Serial Monitor window, which displays the data sent from Arduino to a personal computer. The same way we can send data/command from Serial Monitor to Arduino. The serial communication enables us to control electronic devices connected to Arduino board from a personal computer.
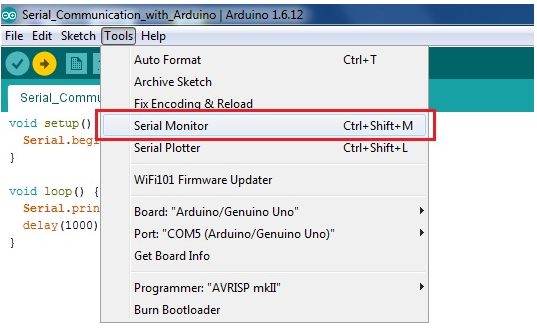
Boards including the Uno, Duemilanove, Diecimila, Nano, and Mega have a chip to convert the hardware serial port on the Arduino chip to Universal Serial Bus (USB) for connection to the hardware serial port.
Example 1. In this example, we will send a string from Arduino to the personal computer. Once we upload the sketch into Arduino, we will see this string printed on Serial Monitor window of Arduino IDE.
void setup() { Serial.begin(9600); } void loop() { Serial.println("Hello from me!"); delay(1000); }
Serial is asynchronous.
It is easy to get caught in the trap of thinking that typing a full word in the Serial Monitor and then clicking Send, means the entire word is sent immediately. However, it is not. Only one character gets sent at a time. Depending on the line ending settings, a few extra characters might be sent automatically.
Asynchronous sending means that to capture multiple character commands requires you to buffer incoming characters. While not difficult, it does tend to start getting complicated.
Data is transmitted and received bit by bit as zeros “0” and ones “1” between Arduino board and personal computer: this is Serial Communication.
Mathematically, a bit is an abstraction used to denote either a logical high or logical low in digital electronics. In reality, the “high” here means anything between 3.3 to 5.0 volts, while logical low refers to 0 volts.
- 1 bit = 0 or 1
- 4 bits = 1 Nibble
- 8 bits = 1 Byte
- 1024 Bytes (8192 Bits) = 1 Kilobyte (KB)
- 1024 Kilobytes (1048576 Bytes) = 1 Megabyte (MB)
- 1024 Megabytes = Gigabyte (GB)
void setup() { Serial.begin(9600); // initiating serial communication at 9600 baud rate. } int count = 0; // initiating a variable name count of data type int to zero void loop() { // tell the serial monitor to print the string data "Number " Serial.print("Number "); // tell the serial monitor to print the current content of the variable count Serial.print(count); // tell the serial monitor to print the string data " is printed on the serial monitor" Serial.println(" is printed on the serial monitor"); // incrementing the content of the variable count by 1 count++; // do not do any other thing until one second has passed delay (1000); }
Explaining the code
Upload the sketch to the Arduino board by clicking the upload button or Ctrl+U. When the sketch is done uploading, click on the tools button, then click on serial monitor, you will see data printing on the serial window every one second.
Example 2. Humans like words, computers like binary: so, just send one character over serial.
This example shows how to control an Arduino board connected via usb interface in order to switch on/off a led.
It’s very simple: when it receives a character through the usb serial connection it switches on the led connected to a pin as output; viceversa, typing another character, the same led is switched off. Every command writes a character on the serial.
A standard Arduino has a single hardware serial port, but serial communication is also possible using software libraries to emulate additional ports (communication channels) to provide connectivity to more than one device. Software serial requires a lot of help from the Arduino controller to send and receive data, so it’s not as fast or efficient as hardware serial.
Serial.available()
Description. Get the number of bytes (characters) available for reading from the serial port. This is data that’s already arrived and stored in the serial receive buffer (which holds 64 bytes).
Returns. The number of bytes available to read.
Serial.begin()
Description. Sets the data rate in bits per second (baud) for serial data transmission. For communicating with Serial Monitor, make sure to use one of the baud rates listed in the menu at the bottom right corner of its screen. You can, however, specify other rates - for example, to communicate over pins 0 and 1 with a component that requires a particular baud rate.
An optional second argument configures the data, parity, and stop bits. The default is 8 data bits, no parity, one stop bit.
Syntax. Serial.begin(speed)
Returns. Nothing
Serial.read()
Description. Reads incoming serial data.
Syntax. Serial.read()
Returns. The first byte of incoming serial data available or -1 if no data is available. Data type:int
Example 3. Arduino Receiving Data.
char num = 0; int ledPin = 13; void setup() { Serial.begin(9600); pinMode(ledPin, OUTPUT); } void loop() { if (Serial.available() > 0) { num = Serial.read() - 48; } if (num == 1) { digitalWrite(ledPin, HIGH); Serial.print("1 was pressed and pin "); Serial.print(ledPin); Serial.println(" is ON"); } else if (num == 2) { digitalWrite(ledPin, LOW); Serial.print("2 was pressed and pin "); Serial.print(ledPin); Serial.println(" is OFF"); } delay(1000); }
char num = 0; int ledPin = 13; void setup() { Serial.begin(9600); pinMode(ledPin, OUTPUT); } void loop() { if (Serial.available() > 0) { num = Serial.read() - 48; } if (num == 1) { digitalWrite(ledPin, HIGH); Serial.print("1 was pressed and pin "); Serial.print(ledPin); Serial.println(" is ON"); } else if (num == 2) { digitalWrite(ledPin, LOW); Serial.print("2 was pressed and pin "); Serial.print(ledPin); Serial.println(" is OFF"); } delay(1000); }
explaining the code
Line 10 code checks if anything was sent from the keyboard. >= means that if something was sent, then that char (character) must be equal to or greater than zero, if nothing was sent, then the Arduino will have to receive anything less than or not equal to 0, which is same as saying nothing was sent.
Line 12 code checks the value stored in the variable num. num will now become the value sent from the keyboard and was read by Arduino. The value that was read is a ‘char’ type of data.
Line 14 code contains an “if statement”, we shall talk about the “if statement” in the future tutorials. However, what the code does is to check “if” the number that was pressed on the keyboard is equal to 1. Note that ‘=’ is not the same thing as ‘==’. The earlier is an assignment operator, while the latter is an equality sign in computer programming. So, if the condition is true, the code in the curly bracket in lines 16 to 19 will be executed.
Line 21 code is another conditional statement function, “else if”, this statement checks if the number pressed on the keyboard is equal to 2. instead of being equal to 1. If it is equal to 2, the code in lines 23 to 26 will be executed.
WORKING
When you upload the code and open the serial monitor console, if you press 1 on your keyboard followed by the enter key, the LED connected to pin 13 of the Arduino will come ON and a text will be displayed on the serial console window telling you that you pressed 1 and pin 13 is ON. If you press 2 on the keyboard followed by the enter key, the LED connected to pin 13 will go OFF, and a text will be displayed on the serial console window also telling you that you pressed 2 and pin 13 is OFF.
Using switch statement.
The switch statement executes code based on the value of a variable. The structure is much cleaner than endless if-else statements and even allows a limited amount of “stacking.”
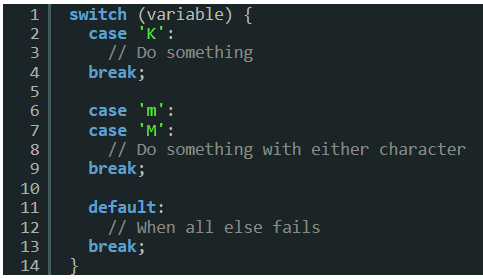
The conditions for the switch-statement are wrapped in curly braces. Each “case” is like saying “if (variable==case).” Inside of the switch block, any code matching the switch case executes and continues to run until the end of the block or a “break” statement.
Stacking Cases.
It is also possible to “stack” case. In the example above both, the characters ‘m’ and ‘M’ will execute the same code. You could also repeat cases if you want multiple actions to happen based on a character.
You must include a “break” at the end of the “case.” The code will not check any more conditions until the next time it sees a “break!”
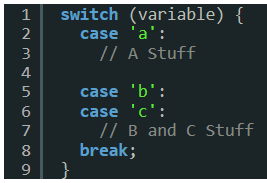
Now just verify and upload the sketch to Arduino and open up Serial Monitor. You are ready to control LED connected PIN 12 of Arduino.
Now just enter ‘a’ (it means ‘acceso’) and press Send button. You will see on board that the LED will turn ON.
When you enter ‘s’ (it means ‘spento’) and hit Send button LED will turn OFF. This is how you can control devices connected to Arduino using a serial interface.
void setup() { Serial.begin(9600); //Set all the pins we need to output pins pinMode(13, OUTPUT); } void loop (){ if (Serial.available()) { //read serial as a character char ser = Serial.read(); //NOTE because the serial is read as "char" and not "int", the read value //must be compared to character numbers //hence the quotes around the numbers in the case statement switch (ser) { case 'a': pinON(13); Serial.println("13;on"); break; case 'b': pinOFF(13); Serial.println("13;off"); break; } } } void pinON(int pin){ digitalWrite(pin, HIGH); } void pinOFF(int pin){ digitalWrite(pin, LOW); }
Note. Try to optimize the memory occupation.

Note that, the character equivalence of the decimal number 48 is zero (0). Also from the ASCII table, you can see that the decimal equivalence of character number 1 is 49. So, if I pressed one(1) on the keyboard, that one(1) is equal to 49 as a char (since we are receiving the data as char) in the sketch, if we write 1 (which is 49 in char) minus 48 (a decimal), we are having 49(char) – 48(decimal) = 1. Hence, the one that we pressed will be displayed as one, instead of 49.
End Of Post